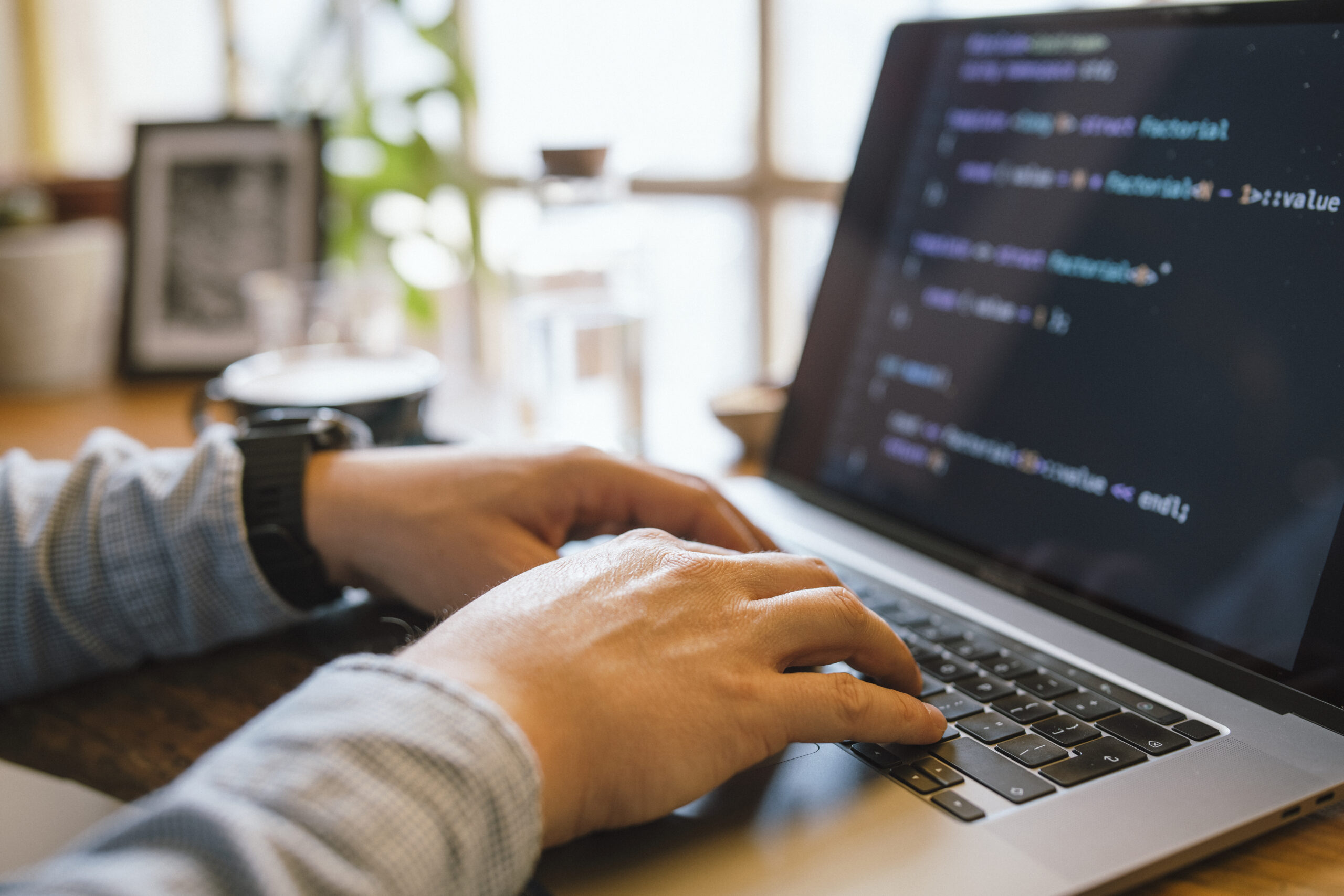
Debugging is The most critical — however usually neglected — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to think methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hrs of stress and substantially increase your productiveness. Allow me to share many approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Applications
On the list of fastest strategies builders can elevate their debugging capabilities is by mastering the instruments they use every day. Whilst creating code is one particular A part of improvement, understanding how you can connect with it correctly all through execution is Similarly important. Fashionable enhancement environments occur Outfitted with highly effective debugging capabilities — but numerous builders only scratch the floor of what these resources can perform.
Acquire, by way of example, an Integrated Development Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code over the fly. When employed correctly, they Enable you to observe just how your code behaves during execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, observe network requests, watch actual-time performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can switch disheartening UI concerns into workable tasks.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage in excess of running processes and memory administration. Discovering these resources could possibly have a steeper Discovering curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Command methods like Git to grasp code heritage, find the exact moment bugs had been released, and isolate problematic variations.
Ultimately, mastering your resources implies heading over and above default options and shortcuts — it’s about producing an personal knowledge of your improvement surroundings to ensure when difficulties occur, you’re not missing at the hours of darkness. The greater you are aware of your tools, the greater time you can spend solving the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the trouble
The most vital — and often ignored — steps in effective debugging is reproducing the condition. Right before leaping to the code or creating guesses, builders will need to make a steady atmosphere or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a game of prospect, generally resulting in squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as possible. Check with queries like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it gets to isolate the precise problems under which the bug happens.
When you’ve gathered sufficient facts, attempt to recreate the condition in your local natural environment. This could signify inputting the identical facts, simulating comparable person interactions, or mimicking system states. If The problem seems intermittently, think about producing automated exams that replicate the sting cases or condition transitions included. These tests not just enable expose the issue and also reduce regressions Later on.
Often, The difficulty might be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Applying resources like virtual equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a mindset. It needs persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more proficiently, take a look at probable fixes safely and securely, and converse additional Plainly with the staff or end users. It turns an summary grievance into a concrete challenge — and that’s where by builders prosper.
Read through and Realize the Error Messages
Error messages are often the most beneficial clues a developer has when a little something goes Completely wrong. In lieu of observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications from the procedure. They generally let you know precisely what happened, wherever it occurred, and occasionally even why it happened — if you understand how to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may possibly lie the genuine root trigger. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can tutorial your investigation and stage you towards the responsible code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can dramatically increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake occurred. Verify relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t ignore compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, aiding you pinpoint concerns speedier, cut down debugging time, and turn into a more efficient and confident developer.
Use Logging Wisely
Logging is Just about the most strong equipment in the developer’s debugging toolkit. When applied correctly, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A superb logging approach begins with figuring out what to log and at what stage. Prevalent logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for basic occasions (like successful start-ups), Alert for likely concerns that don’t break the applying, ERROR for real problems, and Lethal once the method can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure vital messages and slow down your system. Deal with essential occasions, point out alterations, input/output values, and significant selection details with your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to keep track of how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-assumed-out logging method, it is possible to lessen the time it will take to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not only a specialized undertaking—it is a form of investigation. To proficiently identify and repair bugs, developers have to tactic the procedure like a detective solving a mystery. This attitude will help stop working advanced challenges into workable parts and adhere to clues logically to uncover the root result in.
Start off by collecting proof. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain just as much applicable information and facts as you'll be able to without the need of leaping to conclusions. Use logs, exam conditions, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Request your self: What might be causing this behavior? Have any variations recently been made into the codebase? Has this difficulty happened ahead of beneath comparable circumstances? The intention should be to slim down prospects and determine opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a managed surroundings. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code inquiries and let the effects direct you nearer to the truth.
Pay near interest to compact information. Bugs frequently cover inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular mistake, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely understanding it. Momentary fixes may disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for long term troubles and assistance Other individuals fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate systems.
Compose Assessments
Producing checks is one of the most effective approaches to transform your debugging competencies and overall improvement effectiveness. Exams not simply enable capture bugs early but will also function a security Web that gives you self-confidence when creating adjustments to the codebase. A very well-analyzed software is simpler to debug as it means that you can pinpoint particularly where by and when a dilemma takes place.
Get started with device assessments, which center on particular person features or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assistance be sure that a variety of elements of your software get the job done collectively smoothly. They’re specially valuable for catching bugs that happen in elaborate devices with several factors or companies interacting. If one thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what disorders.
Composing checks also forces you to Imagine critically regarding your code. To test a aspect appropriately, you need to be aware of its inputs, anticipated outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you can target correcting the bug and view your examination go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating assessments turns debugging from the disheartening guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s quick to be immersed in the problem—looking at your display for hrs, striving Option after solution. But Just about the most underrated debugging equipment is actually stepping away. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
If you're far too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just several hours previously. In this particular condition, your brain becomes less efficient at trouble-resolving. A brief stroll, a coffee crack, or simply switching to a unique process for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during extended debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Vitality and a clearer way of thinking. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent rule of thumb is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use website that time to maneuver close to, extend, or do a thing unrelated to code. It may sense counterintuitive, Specifically less than tight deadlines, but it surely really causes quicker and more practical debugging In the end.
Briefly, having breaks just isn't an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Just about every Bug
Every bug you come across is much more than simply A short lived setback—It is a chance to improve as a developer. Regardless of whether it’s a syntax error, a logic flaw, or maybe a deep architectural issue, each one can educate you anything precious if you make an effort to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code assessments, or logging? The responses normally expose blind places in the workflow or being familiar with and assist you build much better coding habits going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see designs—recurring problems or typical mistakes—you could proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but people that constantly master from their blunders.
Eventually, Every bug you deal with adds a whole new layer to your skill established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques takes time, follow, and endurance — but the payoff is huge. It would make you a far more efficient, assured, and able developer. Another time you're knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.